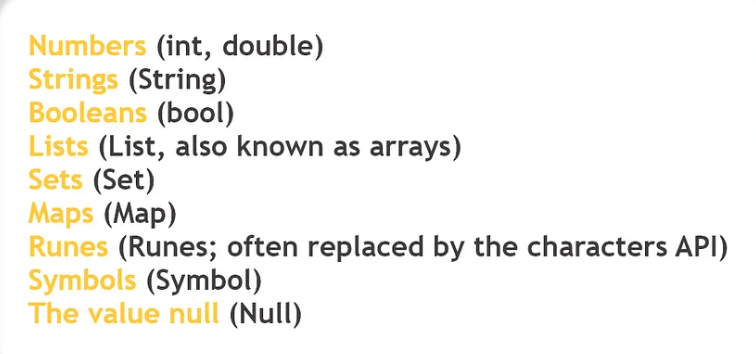
What is Runtime Type?
Runtime type, represented by the runtimeType property, is a feature in Dart that allows developers to obtain the actual type of an object during runtime. Unlike static typing, where types are determined at compile time, runtime type enables dynamic type checking and introspection during program execution.
Why is Runtime Type Useful?
Runtime type provides several benefits in Dart programming:
Dynamic Behavior: Dart is a dynamically-typed language, meaning type checking occurs at runtime. With runtime type, developers can implement dynamic behavior based on the type of objects, enabling more flexible and expressive code.
Type Inspection: Runtime type allows developers to inspect and determine the type of objects dynamically. This is particularly useful when dealing with polymorphic behavior or when the type of objects is not known until runtime.
Debugging and Error Handling: During debugging or error handling scenarios, knowing the runtime type of objects can aid in identifying and resolving issues more effectively.
Using Runtime Type in Dart:
Let’s dive into how we can use runtime type in Dart with a simple example:
void main() {
var object1 = 42;
var object2 = "Hello, Dart!";
print('Runtime type of object1: ${object1.runtimeType}');
print('Runtime type of object2: ${object2.runtimeType}');
}
Output:
Runtime type of object1: int
Runtime type of object2: String